The Workflow Kernel
The class org.imxis.workflow.WorkflowKernel
is the core component of the Imixs-Workflow API. The WorkflowKernel controls the processing life cycle of a process instance (Workitem) according to an Imixs-BPMN process model.
The processing life cycle is defined by a BPMN Event describing the transition between two BPMN Task elements.

The WorkflowKernel is initialized by the Workflowmanager which is providing the WorkflowContext and the BPMN 2.0 Model definition. To process a single Workitem the WorkflowKernel provides the method process():
process(ItemCollection workitem)
The WorkflowKernel controls the following attributes of a process instance:
Attribute | Description |
---|---|
$UniqueID | A unique key to access the process instance |
$WorkitemID | A unique process instance id of this workitem |
$ModelVersion | The Version of the model the workitem belongs to |
$TaskID | The current BPMN Task ID of the workItem |
$lastEvent | The last processed BPMN Event element |
$lastTask | The last assigned BPMN Task element |
The Processing Phase
By calling the method process() from the WorkflowKernel the processing phase for a WorkItem is started. During the processing phase the WorkflowKernel loads the assigned BPMN event and triggers all registered plugins to be executed. During the processing phase additional BPMN Events can be triggered according to the model definition. See the section ‘How to model’ for further details. When the processing phase is completed the WorkflowKernel applies the new BPMN Task element to the workitem as defined by the process model.
Conditional Events
A conditional event is used by the WorkflowKernel to evaluate the output of an event during the processing life-cycle. A conditional-event can be placed before an ExclusiveGateway where each output of the event defines a boolean expression.
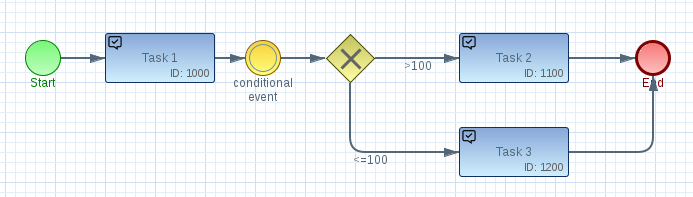
The expressions are evaluated by the WorkflowKernel to compute the output of a BPMN Gateway element. See the section ‘How to model’ for further details about modeling Conditional Events.
Split Events
In Imixs-Workflow a BPMN Event followed by a Parallel Gateway is called a split-event and forces the WorkflowKernel to create a new version of the current process instance.
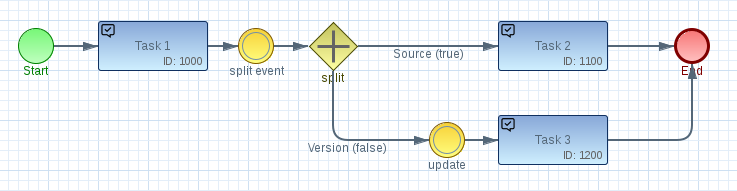
The WorkflowKernel evaluates the conditions assigned to the outcome of the Parallel Gateway. The conditions are either evaluated to the boolean value true or false. If the condition evaluates to ‘true’, this outcome is followed by the current process instance (Source Workitem). If the condition evaluates to ‘false’, then a new version of the Source Workitem is created.
Note: The WorkflowKernel expects that each outcome evaluated to ‘false’ is followed by an Imixs-Event element. This event will be processed by the new created version. If an outcome evaluated to ‘false’ is not followed by an Imixs-Event, a ModelException is thrown. See the section ‘How to model’ for details about modeling Split Events.
Condition | Type | Description |
---|---|---|
true | Source | describes the outcome for the current process instance. |
false | Version | triggers the creation of a new version. |
The current process instance is called the Source Workitem. The Source Workitem and all versions have the same ‘$workitemID’. In addition, the ‘$UniqueID’ of a new version is stored into the attribute ‘$uniqueidVersions’ of the current process instance. A version holds a reference to the Source Workitem in the attribute ‘$uniqueIdSource’.
Attribute | Source | Version | Description |
---|---|---|---|
$workitemId | x | x | A unique shared key across all versions and the source workitem. |
$uniqueIdSource | x | A reference to the $UniqueID of the Source workitem. | |
$uniqueIdVersions | x | A list of $UniqueIDs of all created versions. | |
$created.version | x | Date of creation of a version. | |
$isVersion | x | This temporary attribute indicates that the current instance is a version. |
The temporary attribute ‘$isVersion’ flags the version during the processing phase and can be used by Plugins to handle these workitems. See also the section: Workflow Data.
The WorkflowKernel returns new created versions of the current process instance by the method getSplitWorkitems(). This method is used by the Workflow Engine to store the versions into a database.
Registration of Workflow Plugins
In the processing phase of a WorkItem the WorkflowKernel calls the plug-ins registered by the WorkflowManager. To register a plug-in the WorkflowKernel provides the method :
registerPlugin(String)
Plugins registered by the WorkflowManager will be executed during the processing phase of a workitem. See the section Plugin API for further details about the plugin concept.
The registration of a plugin can be done by an instance of a plugin class or by the class name. Plugins are also supporting the the injection mechanism of CDI.
kernel = new WorkflowKernel(ctx);
// register plugin by name
kernel.registerPlugin(MyPlugin.class.getName());
// register plugin by instance
kernel.registerPlugin(new MyPlugin());
// process workitem
workitem=kernel.process(workitem);
To unregister a single plugin, the method unregisterPlugin() can be called:
// unregister plugin by name
kernel.unregisterPlugin(MyPlugin.class.getName());
The WorkflowContext
The Interface org.imixs.workflow.WorkflowContext defines an abstraction of the workflow management system. The WorkflowContext is used by the WorkflowKernel to provide the runtime-environment information to registered plugins.
The WorkflowContext provides the following methods:
Method | Description |
---|---|
getSessionContext() | returns a session object (e.g. EJB Context or Web Session) |
getModelManager() | returns an instance of the model manager |
The session context is platform specific, for example a surrounding EJB Context, a Web Module or a Spring Context. As the WorkflowKernel is part of the Imixs-Workflow core API it is a platform independent building block. Therefor the WorkflowContext builds a bridge between the Workflow System and the processing engine. For details about the concrete implementation see the WorkflowService implementation.
The Workflow Log
The Imixs WorkflowKernel generates a log entry during each processing phase with information about the current model version, the process entity and the processed workflow event. The log is stored in the property ‘$EventLog’. The log entry has the following format:
ISO8601 Time | Model Version | Process.ActivityID | NewProcessID | comment /optional)
Example:
2014-08-10T16:44:32.025|1.0.0|100.10|100|
2014-08-10T16:45:32.125|1.0.0|100.10|100|
2014-08-10T16:47:12.332|1.0.0|100.20|200|userid|comment
The comment is an optional value which can be provided by a plugin. A comment is stored in the property $eventLogComment
of the current process instance. If no $eventLogComment
attribute is provided the comment section will be empty.